The Honeywell QR reader will be our USB serial device to connect.
Recently I’m working on a project where i need to connect two Honeywell QR readers. The model to connect is the YJHF600 through the usb serial communication with a Raspberry Pi. For this i need to get the QR code scanned using golang as code language.
I will do a reader test of the QR code with the python language because the fastest to code and to prototype with this language
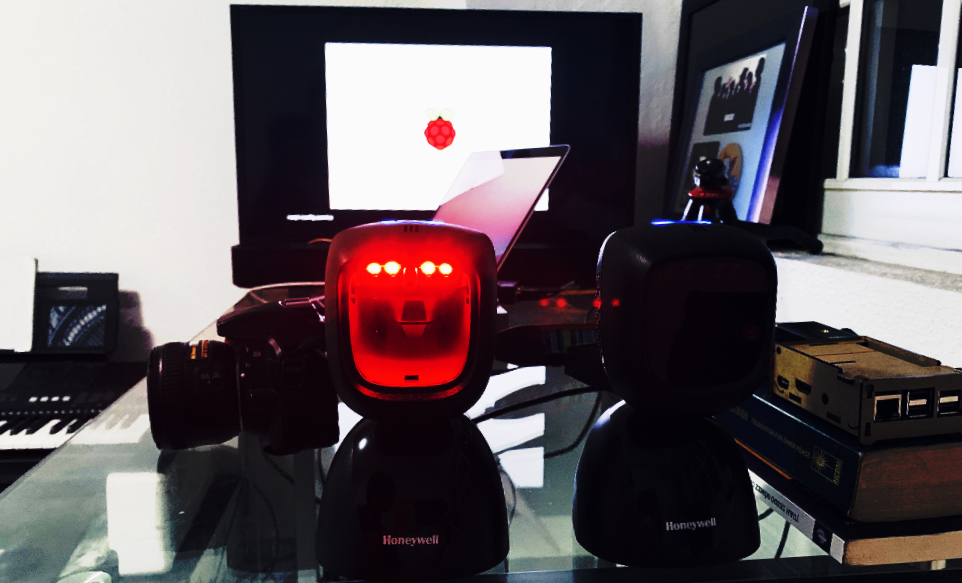
Honeywell QR reader – Raspberry Pi
How the usb communication for Honeyweel readers works?
Like most barcode readers, QR readers often emulate a keyboard and transmit the scanned information as if had been typed. In other words, our device to which we connect the readers usually see them as if they were a qwerty keyboard.
Some readers can be configurated as usb serial communication. This is the case if the Honeywell YJHF600 QR reader. For this reader all we have to do is scan the configuration code provided in the Honeywell reader manual with the QR readers and they will be automatically configured to generate this serial usb communication.
Testing the usb serial commutication between our Raspberry Pi and our Honewell Qr reader
Since we have configured and connected our serial usb device on the Raspberry Pi, we execute the command
dmesg | grep tty
.
This command should return a response like this:
[ 0.000000] Kernel command line: coherent_pool=1M 8250.nr_uarts=1 bcm2708_fb.fbwidth=1360 bcm2708_fb.fbheight=768 bcm2708_fb.fbswap=1 smsc95xx.macaddr=B8:27:EB:F4:94:87 vc_mem.mem_base=0x3ec00000 vc_mem.mem_size=0x40000000 console=tt1 root=PARTUUID=6c586e13-02 rootfstype=ext4 elevator=deadline fsck.repair=yes rootwait quiet splash plymouth.ignore-serial-consoles [ 0.000297] console [tty1] enabled [ 0.824827] 3f201000.serial: ttyAMA0 at MMIO 0x3f201000 (irq = 81, base_baud = 0) is a PL011 rev2 [ 0.826467] 3f215040.serial: ttyS0 at MMIO 0x0 (irq = 53, base_baud = 31250000) is a 16550 [ 2.936625] systemd[1]: Created slice system-getty.slice. [ 6.548674] cdc_acm 1-1.1.3:1.0: ttyACM0: USB ACM device [47913.774867] cdc_acm 1-1.1.2:1.0: ttyACM1: USB ACM device
There are two possible ways to detect your device. One is as ttyACM and the other is as ttyUSB,. Fort this test with the Honeywell QR reader they are detected as ttyACM1 and ttyACM2
[ 6.548674] cdc_acm 1-1.1.3:1.0: ttyACM0: USB ACM device [47913.774867] cdc_acm 1-1.1.2:1.0: ttyACM1: USB ACM device
Now that we know how to call our Honeyweel reader we can make a QR reader test just with this command:
sudo cat /dev/ttyACM0
Any QR code that we pass through the scanner of our Honeywell reader will show us its value in the output of this command. The same happens for the second reader if we vary ttyACM0 by ttyACM1 in the command.
Working with Python and the Honeywell QR readers
Reading the values returned by a device that can establish usb serial communication is really easy in Linux as we could see in the previous command.
In the case of Python it is enough to analyze the buffer of our /dev/ttyACM0 and /dev/ttyACM1 in separate threads to obtain the codes scanned by our Honeywell QR readers as in this example code:
import os, sys import serial import time import _thread def readSerialOne(Thread): ser = serial.Serial('/dev/ttyACM0', 19200, timeout = 0) while True: line = ser.readline().decode() if len(line) > 0: print(line) time.sleep(0.1) def readSerialTwo(Thread): ser = serial.Serial('/dev/ttyACM1', 19200, timeout = 0) while True: line = ser.readline().decode() if len(line) > 0: print(line) time.sleep(0.1) try: _thread.start_new_thread( readSerialOne, ("QR-1", ) ) _thread.start_new_thread( readSerialTwo, ("QR-2", ) ) except: print ("Error: unable to start thread") while 1: pass
To execute the above code is only required to install the library pyserial. This library could be installed with the following command
sudo apt-get install python3-pip sudo pip3 install pyserial
Finally we just run our code with python
sudo python3 usb-serial-reader.py
Now with this code running any QR code scanned in any of the two Honeywell QR readers will be visualized in our terminal.
Link to github for this python usb serial communication example.
Clic aquí para visualizar está entrada en su versión en español.
Leave a Comment